Browserify is a bundler that magically normalizes all your npm
requirements into something a browser can understand. This means that your whole application can be minified into just 1 script that you can include in a regular HTML page.
Let’s start with the basics. Imagine you have your application written in React, or maybe even in jQuery, and now you want to make sure it’s built into one single script, minified and compressed of course. This is where Browserify comes handy.
Example .jsx
Let’s imagine we have a simple ticking clock application. All it does is displays the date, and it uses Preact to handle re-rendering on state changes.
import { h, render, Component } from 'preact';
import React from 'preact';
class Foo extends Component {
state = { tick: new Date() }
componentDidMount() {
// update time every second
this.timer = setInterval(() => {
this.setState({ tick: new Date() });
}, 1000);
}
render() {
return <div>
{this.state.tick.toLocaleString()}
</div>
}
}
const dom = document.getElementById('root');
render(<Foo />, dom);
The script assumes that there’s a <div id="root"></div>
somewhere on the page, and then renders our preact component to that element. Everything is in main.jsx, works fine and all, but now you want to build it into something that you can distribute or include in a page online.
Require Browserify, Babelify and Babel presets
npm i --save-dev @babel/core @babel/preset-env @babel/preset-react babel-loader babelify browserify
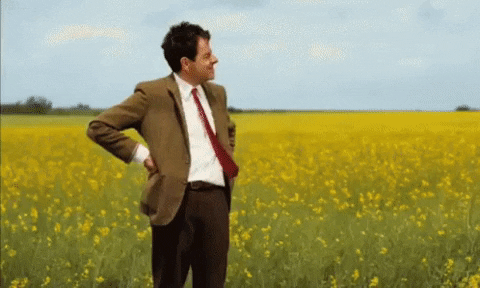
After some time waiting, we now have all required modules.
Babel presets and build script
Next step is to add babel presets to our package.json
:
"babel": {
"presets": [
"@babel/preset-env",
"@babel/preset-react"
]
},
Those presets will manage all the syntax transforms, as well as process JSX files for you.
After this, we only need to add our build line into package.json’s scripts section:
"scripts": {
"build": "browserify main.jsx --transform babelify --standalone util > main.min.js"
}
Back to our command line..
$ npm run build
> preact@1.0.0 build blablabla/codewithnodejs/preact
> browserify main.jsx --transform babelify --standalone util > main.min.js
(This is the moment where you pray to not have any errors during the build process)
Main.min.js can now be included in HTML
And now, the culmination of the process: include the main.min.js
script in our HTML.
<div id="root"></div>
<script src="main.min.js"></script>
If everything went well, you should get a ticking date display!
Did you know that I made an in-browser image converter, where you can convert your images without uploading them anywhere? Try it out and let me know what you think. It's free.